Introducción:
En este código de ejemplo te mostraré cómo podemos averiguar el handle (o
manejador que dicen algunos) de una ventana según en la posición en la que
se encuentre el puntero (o cursor) del ratón.
Una vez que sepamos el handle de la ventana a la que señala el ratón (o
mouse) capturaremos el texto de esa ventana y lo mostraremos además del
handle, todo esto a título informativo, ya que en realidad de utilidad tiene
poco, pero al menos nos servirá para saber cómo usar las funciones del API de Windows
WindowFromPoint y GetWindowText, además de
la clase Cursor.Position del propio .NET Framework (y
definida en el espacio de nombres System.Drawing), ésta
última la utilizo en lugar de la función del API GetCursorPos,
ya que son equivalentes.
Por ahora te dejo el código de Visual Basic .NET (el ejemplo lo he hecho
con el Visual Studio 2010, pero en teoría es válido para cualquier versión
que sea igual o superior a Visual Basic 2005.
Cuando ponga el código de C# (lo mismo ya está puesto el código y se me ha olvidado
borrar este comenterio) también será válido para cualquier versión de ese
lenguaje, aunque todos los que sean anteriores al incluído con Visual Studio
2005 tendrás que comprobarlo por tu propia cuenta y riesgo... jejeje
Para usar este código debes tener un formulario con los siguientes
controles:
dos etiquetas, dos cajas de texto (txtTexto y txtHandle), un botón
(btnCerrar) y un timer (timer1), tal como se muestra en la siguiente captura:
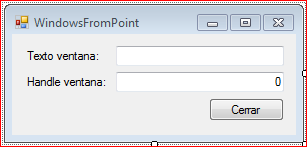
El código de ejemplo para Visual Basic .NET
'------------------------------------------------------------------------------
' Ejemplo de uso del API WindowFromPoint (21/Dic/10)
'
' ©Guillermo 'guille' Som, 2010
'------------------------------------------------------------------------------
Imports vb = Microsoft.VisualBasic
Imports System
Imports System.Windows.Forms
Imports System.Drawing
Imports System.Runtime.InteropServices
Imports System.Text
Public Class Form1
' Las funciones del API y otras que las usan
' Obtener la ventana según la posición indicada
<System.Runtime.InteropServices.DllImport("user32.dll")> _
Private Shared Function WindowFromPoint(ByVal point As System.Drawing.Point) As Integer
End Function
' Obtener el texto de la ventana indicada por el handle
<System.Runtime.InteropServices.DllImport("user32.dll")> _
Private Shared Function GetWindowText(ByVal hWnd As Integer, _
ByVal lpString As System.Text.StringBuilder, _
ByVal cch As Integer) As Integer
End Function
Private Function GetText(ByVal hWnd As Integer) As String
Dim titulo As New System.Text.StringBuilder(New String(" "c, 256))
Dim ret As Integer
Dim nombreVentana As String
ret = GetWindowText(hWnd, titulo, titulo.Length)
nombreVentana = vb.Left(titulo.ToString, ret)
Return nombreVentana
End Function
Private Sub btnCerrar_Click(ByVal sender As System.Object, _
ByVal e As EventArgs) Handles btnCerrar.Click
Me.Close()
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, _
ByVal e As EventArgs) Handles MyBase.Load
timer1.Interval = 300
timer1.Enabled = True
End Sub
Private Sub timer1_Tick(ByVal sender As System.Object, _
ByVal e As EventArgs) Handles timer1.Tick
timer1.Enabled = False
Dim p As System.Drawing.Point = System.Windows.Forms.Cursor.Position
Dim h As Integer = WindowFromPoint(p)
If h <> 0 Then
If h = Me.Handle.ToInt32 Then
' si es este formulario
' no mostrar nada y salir
' (aunque puede ser un control en vez del formulario)
timer1.Enabled = True
Exit Sub
End If
' obtener el texto de la ventana según el handle
txtTexto.Text = GetText(h)
txtHandle.Text = h.ToString
End If
timer1.Enabled = True
End Sub
End Class
El código de ejemplo para Visual C#
public static class UtilAPI
{
// Las funciones del API y otras que las usan
// Obtener la ventana seg\f1ú\f0 n la posición indicada
[System.Runtime.InteropServices.DllImport("user32.dll")]
public extern static int WindowFromPoint(System.Drawing.Point point);
// Obtener el texto de la ventana indicada por el handle
[System.Runtime.InteropServices.DllImport("user32.dll")]
private extern static int GetWindowText(
int hWnd,
System.Text.StringBuilder lpString,
int cch);
public static string GetText(int hWnd)
{
System.Text.StringBuilder titulo =
new System.Text.StringBuilder(new string(' ', 256));
int ret;
string nombreVentana;
ret = GetWindowText(hWnd, titulo, titulo.Length);
nombreVentana = titulo.ToString().Substring(0, ret);
return nombreVentana;
}
}
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
timer1.Interval = 300;
timer1.Enabled = true;
}
private void btnCerrar_Click(object sender, EventArgs e)
{
this.Close();
}
private void timer1_Tick(object sender, EventArgs e)
{
timer1.Enabled = false;
System.Drawing.Point p = System.Windows.Forms.Cursor.Position;
int h = UtilAPI.WindowFromPoint(p);
if (h != 0)
{
if (h == this.Handle.ToInt32())
{
// si es este formulario
// no mostrar nada y salir
// (aunque puede ser un control en vez del formulario)
timer1.Enabled = true;
return;
}
// obtener el texto de la ventana según el handle
txtTexto.Text = UtilAPI.GetText(h);
txtHandle.Text = h.ToString();
}
timer1.Enabled = true;
}
}
Y esto es todo... ya sabes...
Nos vemos.
Guillermo